Zebrafy
Zebrafy is a Python 3 library for converting PDF and images to and from Zebra Programming Language (ZPL) graphic fields (^GF).
Zebrafy consists of three conversion tools:
ZebrafyImage — convert an image into valid ZPL
ZebrafyPDF — convert a PDF into valid ZPL
ZebrafyZPL — convert valid ZPL graphic fields into images or PDF
If you want more control over the resulting ZPL data, ZebrafyImage and ZebrafyPDF support the following optional parameters:
Parameter |
Description |
---|---|
|
ZPL graphic field format type (default
|
|
Invert the black and white in the image/PDF output. ( |
|
Dither the result instead of hard limit on black pixels. ( |
|
Black pixel threshold for image without dithering ( |
|
Width of the image in the resulting ZPL, |
|
Height of the image in the resulting ZPL, |
|
Pixel x position of the graphic field in resulting ZPL (default |
|
Pixel y position of the graphic field in resulting ZPL (default |
|
Add ZPL header and footer or only get the ZPL graphic field output ( |
Getting Started
Installation
pip install zebrafy
Dependencies
Pip handles all dependencies automatically. This library is built on top of:
Example Usage
Image to ZPL Graphic Field with ZebrafyImage
Convert image bytes into a complete ZPL string and save to file:
from zebrafy import ZebrafyImage
with open("source.png", "rb") as image:
zpl_string = ZebrafyImage(image.read()).to_zpl()
with open("output.zpl", "w") as zpl:
zpl.write(zpl_string)
Example usage with optional parameters:
from zebrafy import ZebrafyImage
with open("source.png", "rb") as image:
zpl_string = ZebrafyImage(
image.read(),
format="Z64",
invert=True,
dither=False,
threshold=128,
width=720,
height=1280,
pos_x=100,
pos_y=100,
complete_zpl=True,
).to_zpl()
with open("output.zpl", "w") as zpl:
zpl.write(zpl_string)
Alternatively, ZebrafyImage also accepts PIL Image as the image parameter instead of image bytes:
from PIL import Image
from zebrafy import ZebrafyImage
pil_image = Image.new(mode="RGB", size=(100, 100))
zpl_string = ZebrafyImage(pil_image).to_zpl()
with open("output.zpl", "w") as zpl:
zpl.write(zpl_string)
PDF to ZPL Graphic Field with ZebrafyPDF
Convert PDF bytes into a complete ZPL string and save to file:
from zebrafy import ZebrafyPDF
with open("source.pdf", "rb") as pdf:
zpl_string = ZebrafyPDF(pdf.read()).to_zpl()
with open("output.zpl", "w") as zpl:
zpl.write(zpl_string)
ZebrafyPDF conversion supports the same optional parameters as ZebrafyImage conversion:
from zebrafy import ZebrafyPDF
with open("source.pdf", "rb") as pdf:
zpl_string = ZebrafyPDF(
pdf.read(),
format="Z64",
invert=True,
dither=False,
threshold=128,
width=720,
height=1280,
pos_x=100,
pos_y=100,
complete_zpl=True,
).to_zpl()
with open("output.zpl", "w") as zpl:
zpl.write(zpl_string)
ZPL to PDF or Images with ZebrafyZPL
Convert all graphic fields from a valid ZPL file to PIL Images and save to image files:
from zebrafy import ZebrafyZPL
with open("source.zpl", "r") as zpl:
pil_images = ZebrafyZPL(zpl.read()).to_images()
for count, pil_image in enumerate(pil_images):
pil_image.save(f"output_{count}.png", "PNG")
Convert all graphic fields from a valid ZPL file to PDF bytes and save to PDF file:
from zebrafy import ZebrafyZPL
with open("source.zpl", "r") as zpl:
pdf_bytes = ZebrafyZPL(zpl.read()).to_pdf()
with open("output.pdf", "wb") as pdf:
pdf.write(pdf_bytes)
Contributing and Issues
Contributions and bug reports are welcome and can be submitted on the GitHub page.
The project does not yet have a well-defined scope, and I’m open to new feature requests. Features currently in consideration are:
HTML to ZPL conversion by implementing standard HTML elements into ZPL commands
Extract text from a PDF to render it as a native ZPL command instead of graphic field
License
This source is released under the GNU Lesser General Public License v3.0.
Logo
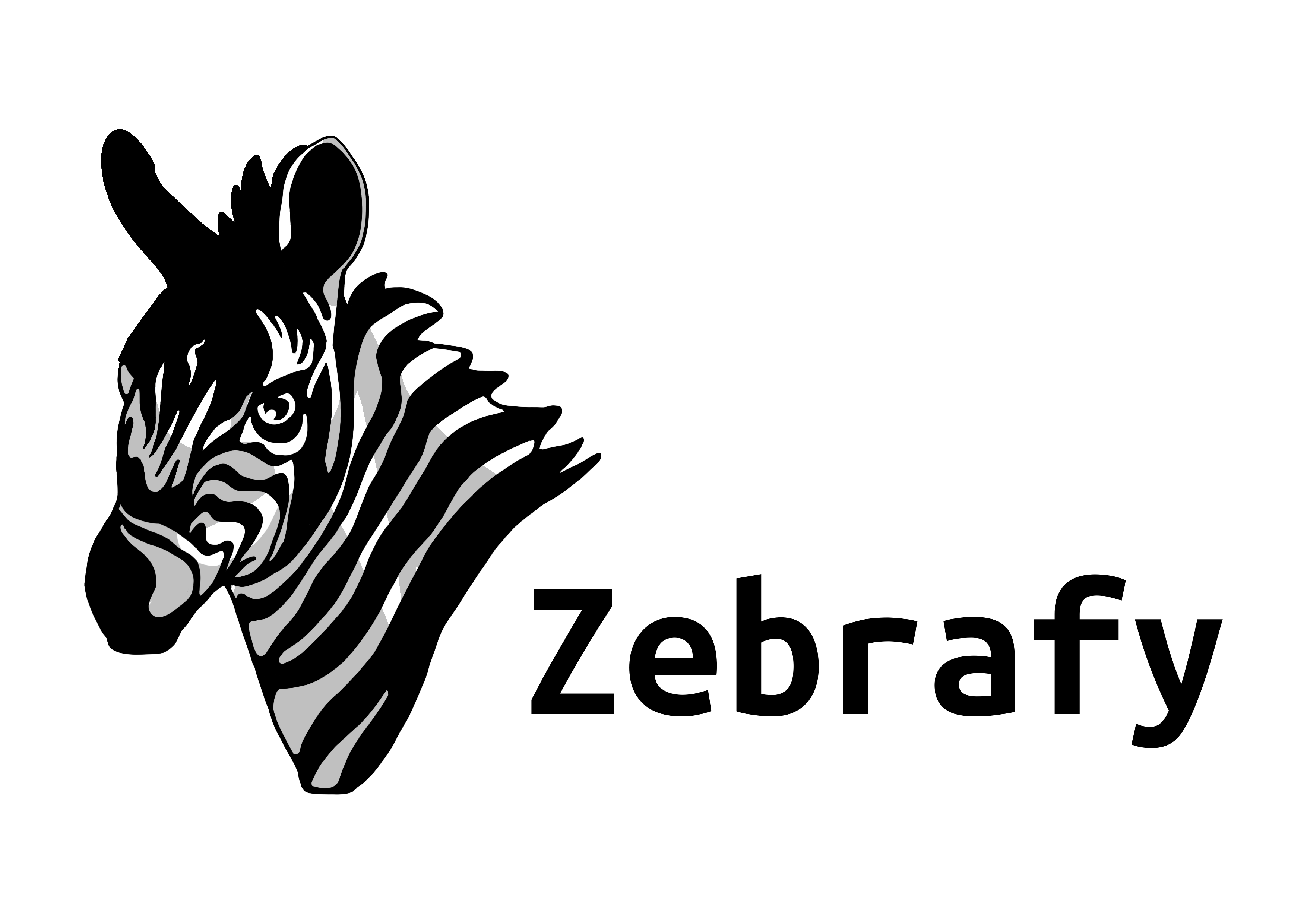
Note
This project is under active development.